## Simple Hackable Pomodoro Timer
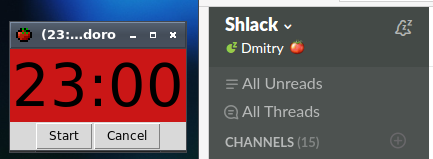
There are tons Pomodoro timers out there. But they are either not customizable, or have "Enterprise Architecture" with multiple methods and calls in the source code, making it complicated to quickly make changes. What if want to quickly customize timer for yourself?
You may want to:
* Score Habitica habits on completed or canceled Pomodoros
* Set Slack to "do not disturb" mode while Pomodoro is running
* Set Tomato Emoji as Slack status, so your colleagues get that you are "trying to concentrate"
* Update Beeminder to match goal of completing *n* Pomodoros per day
* Change color to match your favorite breed of tomato
* ...
So I've created [one more Pomodoro timer](https://github.com/dmi3/tomatych). Less than 50 lines of *very simple cross-platform[^tk] Python script. By default, it displays "always on top" window with a timer in window and title.
Source on Github
The code has no options, as its self-explanatory and intended to be hacked and modified to fit your specific vision of how Pomodoro timers should work.
### Recipes
#### Score tasks in Habitica
* Get [USER_ID and API_TOKEN](https://habitica.com/#/options/settings/api)
* Set `TASK_ID` = [Task Alias](http://habitica.wikia.com/wiki/Task_Alias)
* In `stop` and following line:
```python
requests.post('https://habitica.com/api/v3/tasks/'+TASK_ID+'/score/down', headers={'x-api-key': API_TOKEN, 'x-api-user': USER_ID})
```
* In `complete` add following line:
```python
requests.post('https://habitica.com/api/v3/tasks/'+TASK_ID+'/score/up', headers={'x-api-key': API_TOKEN, 'x-api-user': USER_ID})
```
#### Set Do Not Disturb in Slack
* Get [xoxp_TOKEN](https://api.slack.com/custom-integrations/legacy-tokens)
In `start` add following line:
```python
requests.get('https://slack.com/api/dnd.setSnooze', params=(('token', xoxp_TOKEN), ('num_minutes', '25')))
```
* In `complete` and `cancel` add following line:
```python
requests.get('https://slack.com/api/dnd.endSnooze', params=(('token', xoxp_TOKEN),))
```
#### Set Slack status to Tomato Emoji
* In `start` add following line:
```python
requests.post('https://slack.com/api/users.profile.set', params=(('token', xoxp_TOKEN), ('name','status_emoji'), ('value', ':tomato:')))
```
* In `complete` and `cancel` add following line:
```python
requests.post('https://slack.com/api/users.profile.set', params=(('token', xoxp_TOKEN), ('name','status_emoji')))
```
#### Play sound on completion
* Install vlc.
* In `complete` add following line:
```python
os.system("cvlc /path/to/sound.wav vlc://quit")
# or
os.system("paplay /path/to/sound.wav vlc://quit")
```
#### Set Taskbar icon
* Credit goes to [isaiah658](https://openclipart.org/detail/215656/pixel-tomato).
* In `__init__` add:
```python
self.root.tk.call('wm', 'iconphoto', self.root._w, tk.PhotoImage(data="R0lGODlhIAAgAOMIAAAAAHkAAJcDALUhBgBlANM/JAChAPFdQv///////////////////////////////yH+EUNyZWF0ZWQgd2l0aCBHSU1QACH5BAEKAAgALAAAAAAgACAAAASwEMlJq704622BB0ZofKDIUaQ4fuo5pSIcupK8eu1GkkM/EEDC7oMZeny/oBFQNCKDQmNz+FRKX5+D9lDoFlRIsG+55XrFPfSAvPV+RWH42Fh2q9VLN3LPHwj+AnlefYR+gIJdhX2AgUZ6inuMS5CGjH8BmAGTkJaAmZpOnJ0Cn5uKo6SZJCgfSKilRBc8Pq+qsR2ttKOwHlMArru2vTpLVy7FxifIQzQIyzvN0dLTEhEAOw=="))
```
[^tk]: And thats kids, how we oldtimers created cross-paltform apps before your fancy node and electron was invented.
🏷️Minimalism 🏷️Api